LinkInPurry Jobs
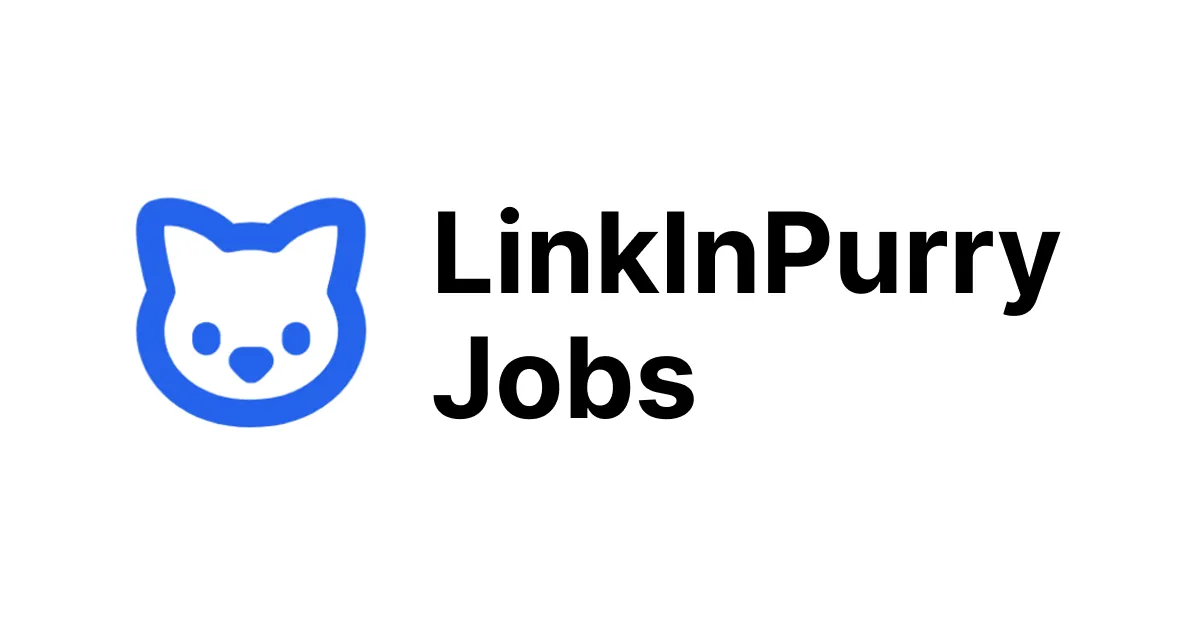
Description
LinkInPurry is a job market platform that serves as a bridge between job seekers and companies, offering a streamlined recruitment process. As part of IF3110 Web-Based Development coursework, this project served as the first assignment to demonstrate web development fundamentals.
Library / Tools Used
- Vanilla HTML , CSS , JavaScript (Front-End)
- Vanilla PHP (Back-End)
- PostgreSQL (Database)
- Quill (Rich Text Editor)
- Docker (Containerization)
Features
- Landing Page
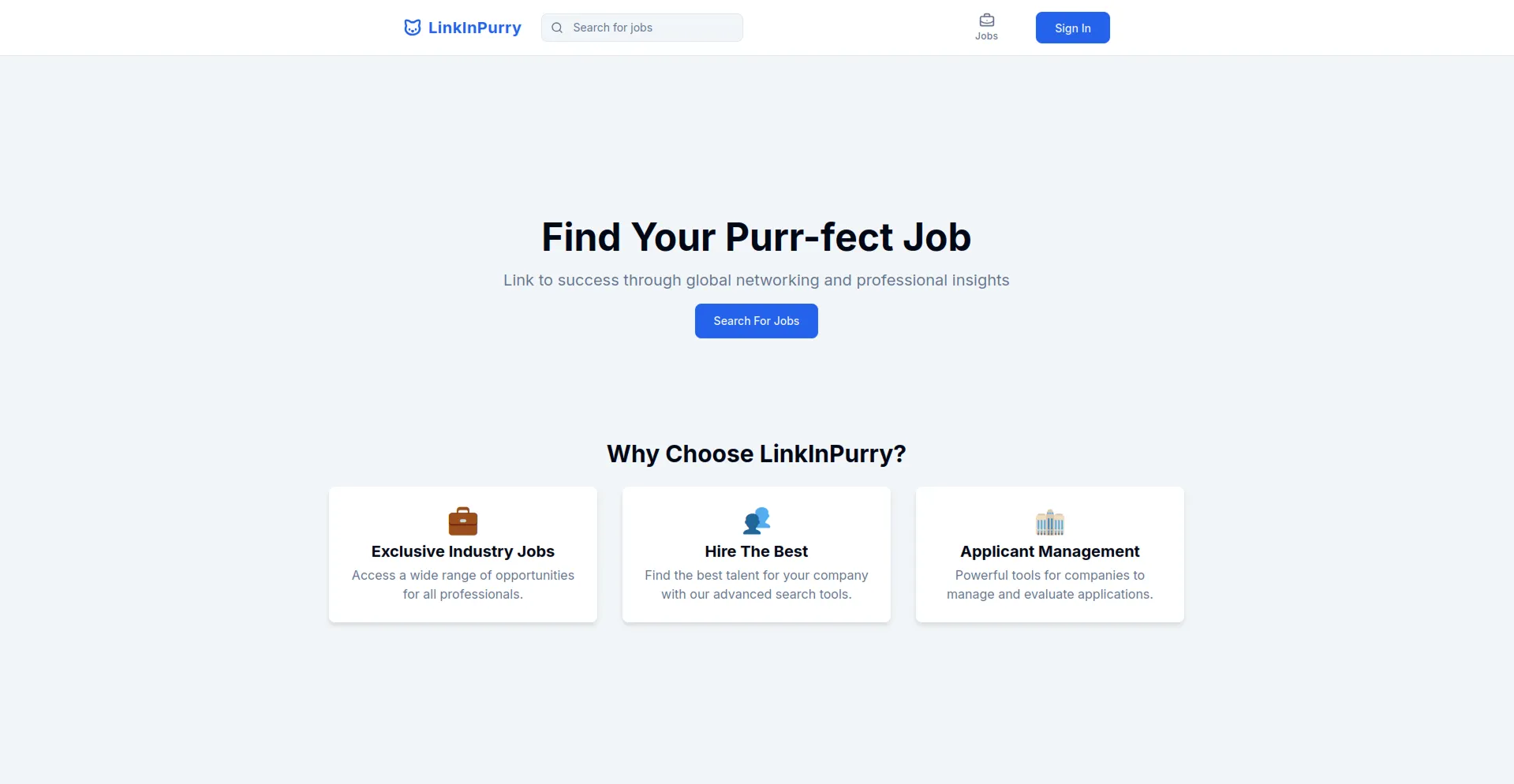
- Job Search Page (Job Seeker)
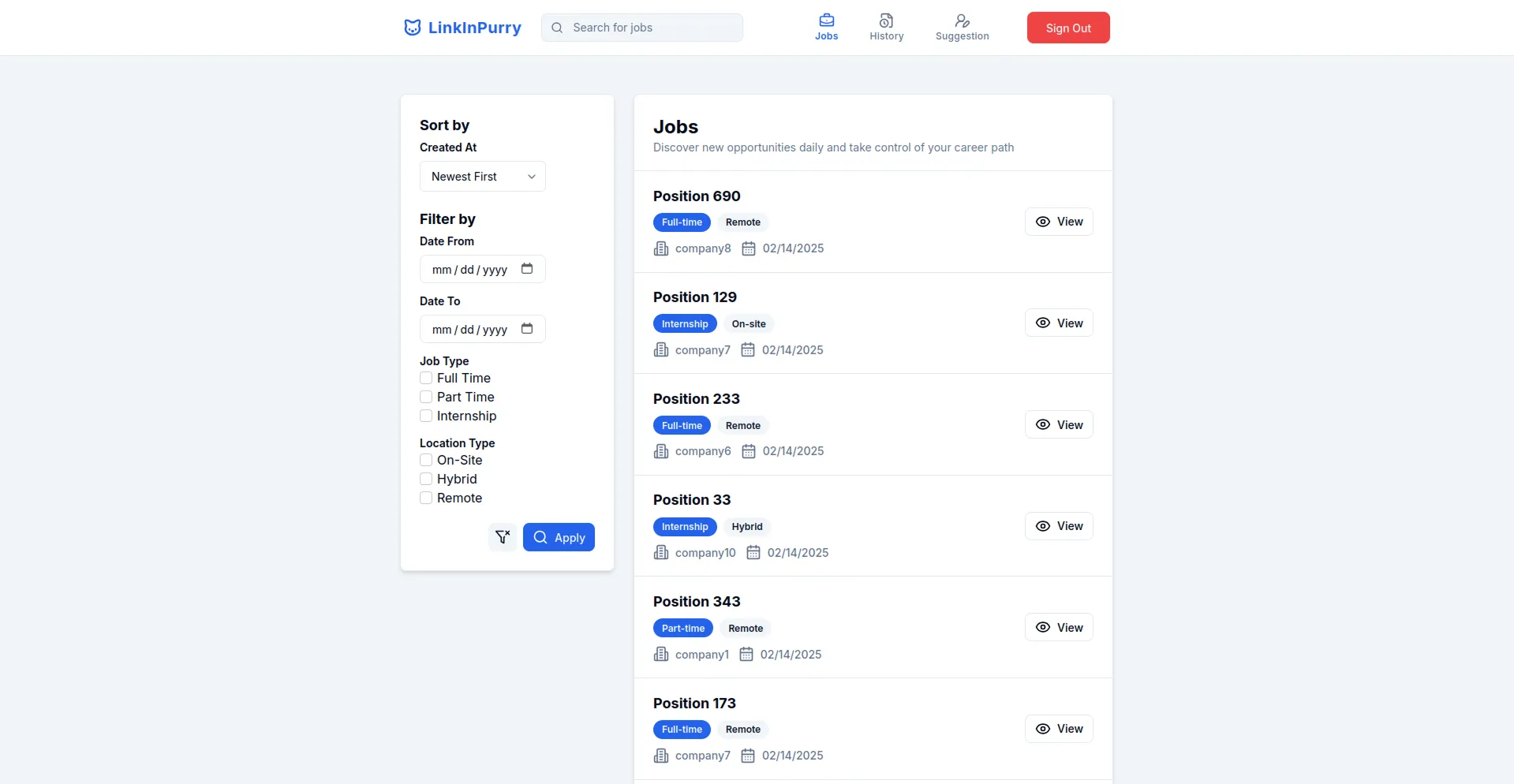
- Job Detail Page
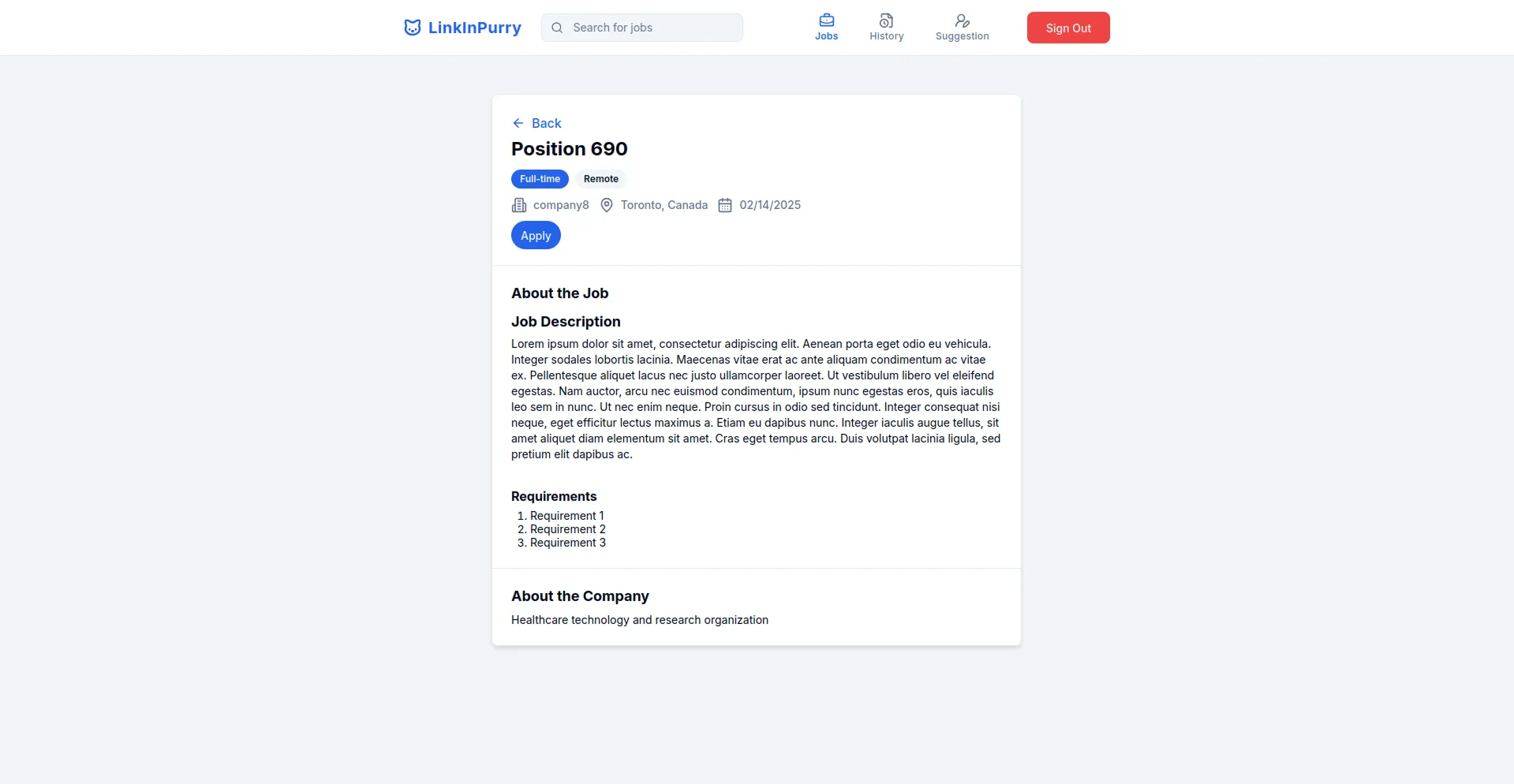
- Job Application History Page (Job Seeker)
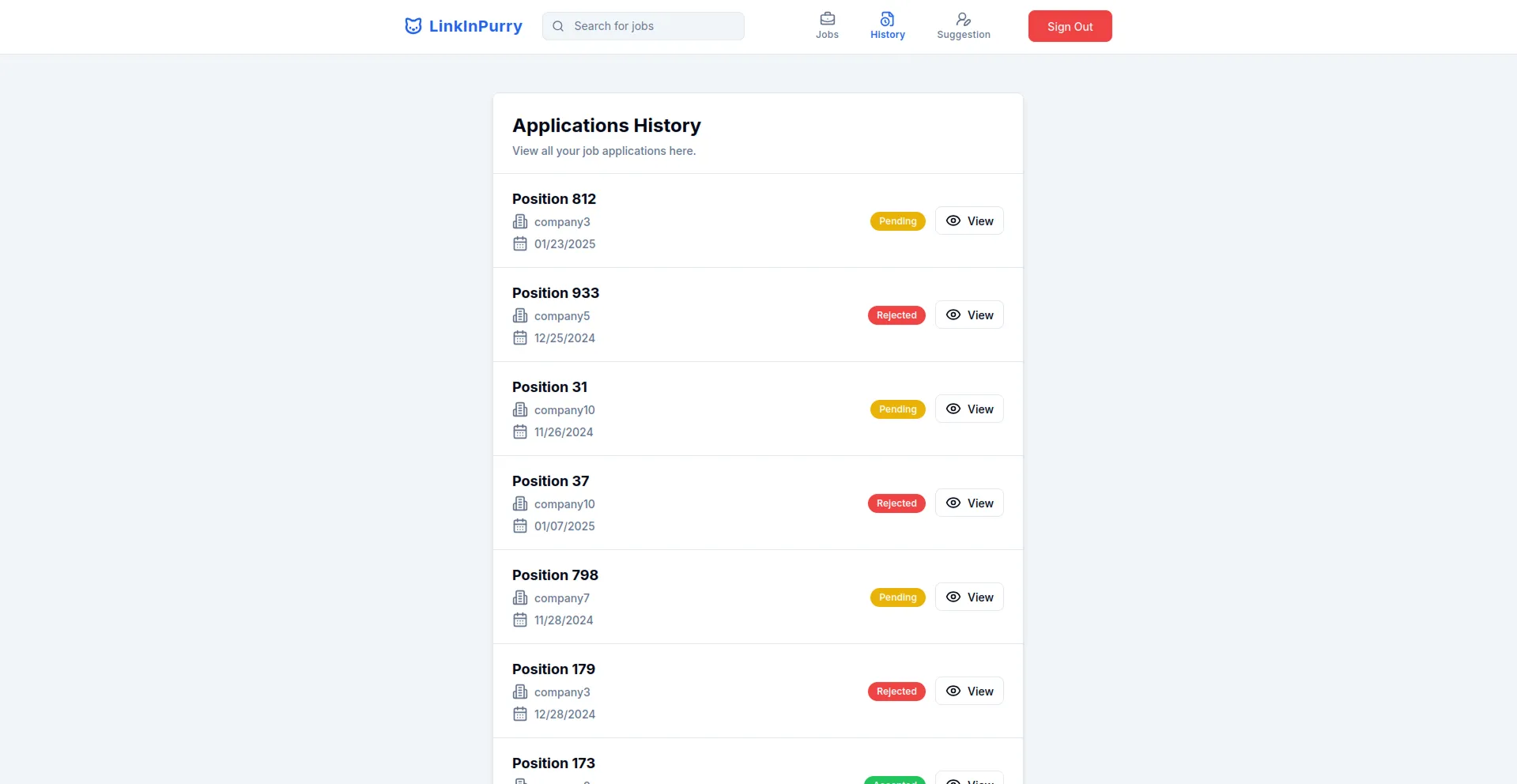
- Job Suggestion Page (Job Seeker)
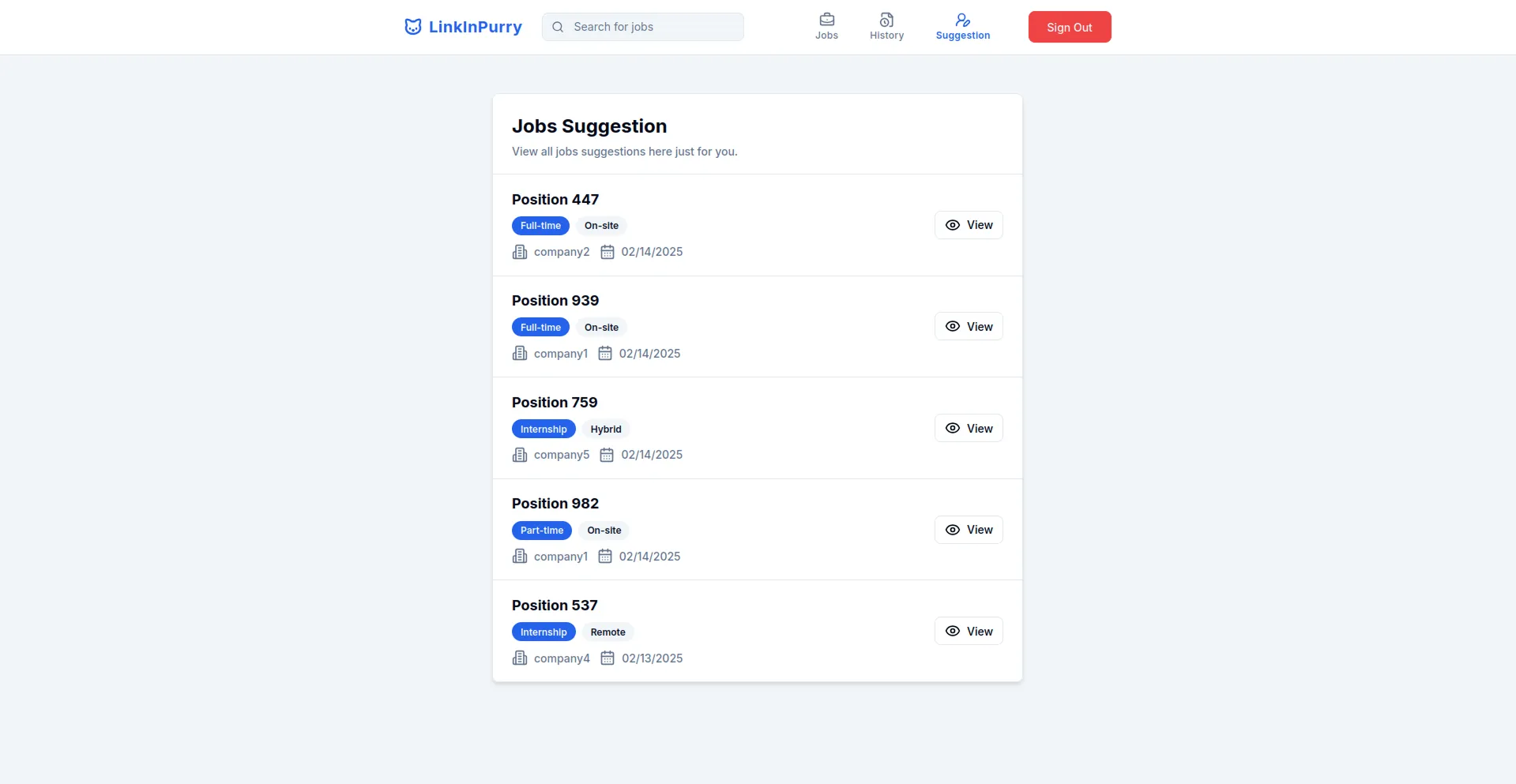
- Job Listing Page (Company)
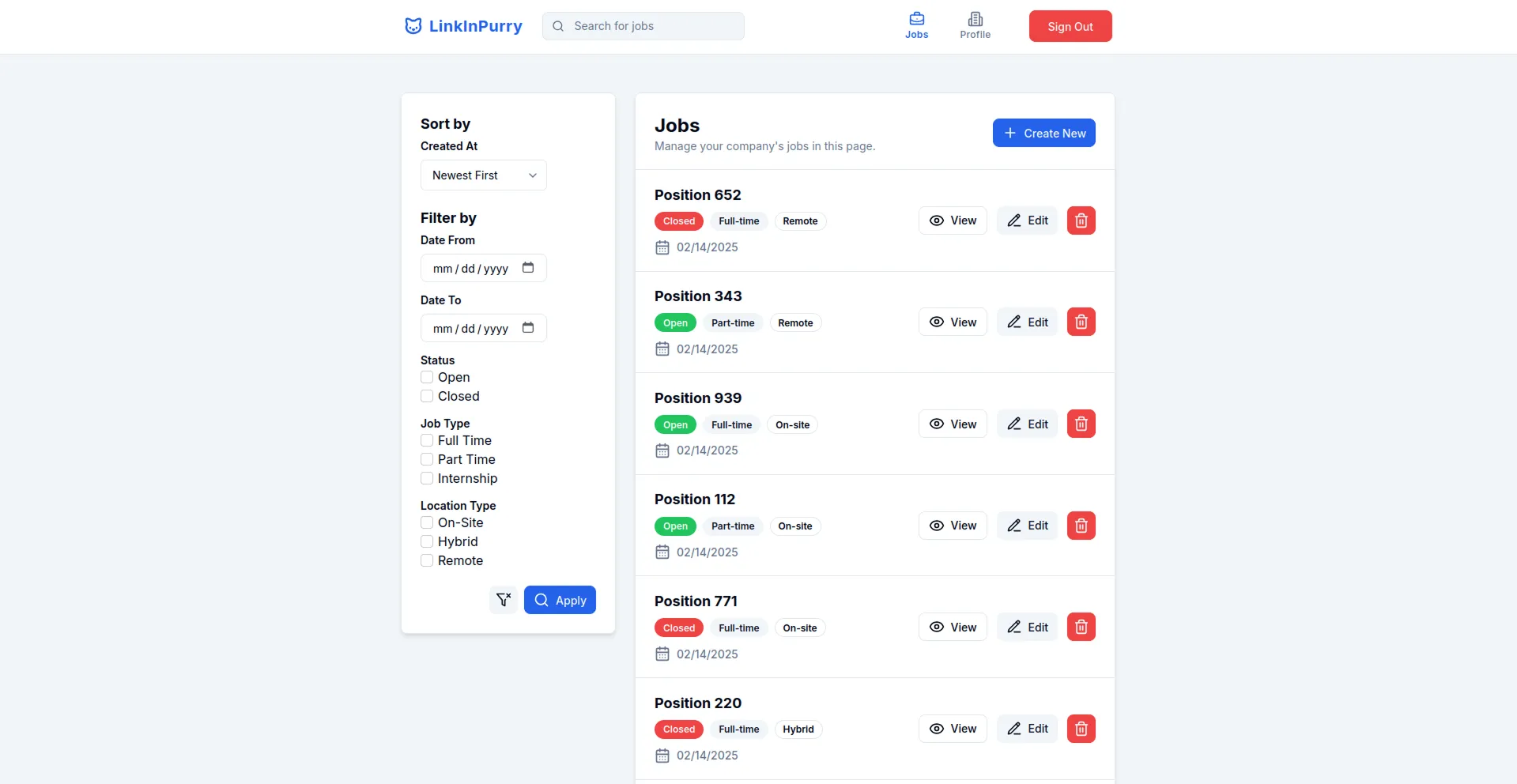
- Job Applications List Page (Company)
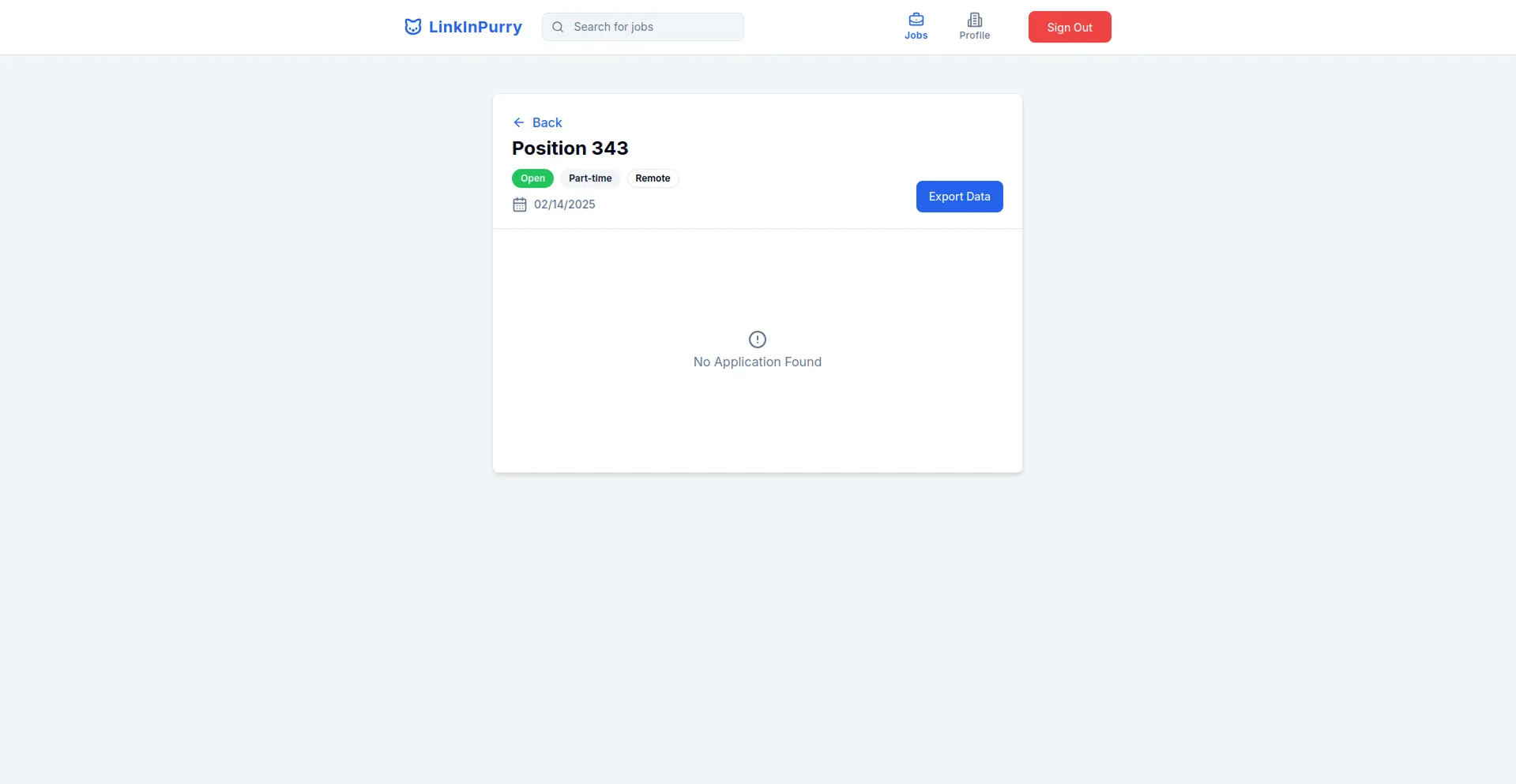
- Job Application Review Page (Company)
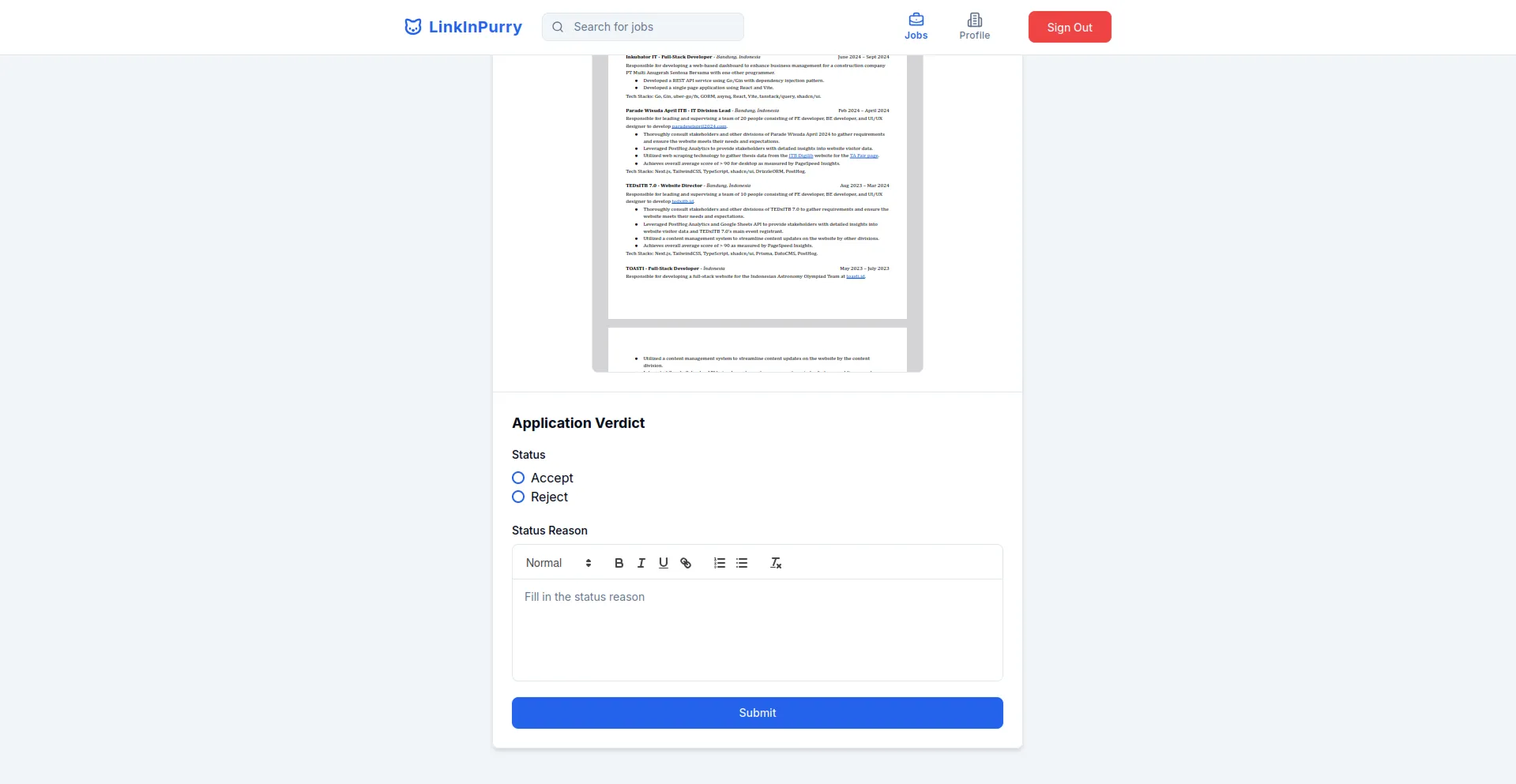
Development Experience
My recent experience with vanilla PHP was quite enlightening. I discovered that vanilla PHP requires careful attention to security measures, as it's particularly vulnerable to common web security threats like XSS and CSRF attacks. Security implementation demands meticulous consideration of various aspects. For instance, XSS prevention requires converting special characters to HTML entities to ensure user input cannot be executed as malicious HTML or JavaScript.
xss.php
<?php
// Simple form to demonstrate XSS protection
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$userInput = $_POST['message'] ?? '';
// Convert special characters to HTML entities
$safeOutput = htmlspecialchars($userInput, ENT_QUOTES, 'UTF-8');
}
?>
<!DOCTYPE html>
<html>
<body>
<!-- Input Form -->
<form method="POST">
<input type="text" name="message" placeholder="Enter some text">
<button type="submit">Submit</button>
</form>
<!-- Display the input -->
<?php if (isset($safeOutput)): ?>
<h3>Your input (safely displayed):</h3>
<div><?php echo $safeOutput; ?></div>
<?php endif; ?>
</body>
</html>
CSRF protection necessitates implementing token generation and verification for all form submissions.
csrf.php
<?php
// Start session at the beginning of your script
session_start();
// Generate CSRF token if it doesn't exist
if (!isset($_SESSION['csrf_token'])) {
$_SESSION['csrf_token'] = bin2hex(random_bytes(32));
}
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
if (!isset($_POST['csrf_token']) || $_POST['csrf_token'] !== $_SESSION['csrf_token']) {
die('CSRF token validation failed');
}
// If we get here, CSRF validation passed
echo 'Form submitted successfully!';
}
?>
<!DOCTYPE html>
<html>
<body>
<form method="POST" action="">
<!-- Include CSRF token as hidden field -->
<input type="hidden" name="csrf_token" value="<?php echo $_SESSION['csrf_token']; ?>">
<input type="text" name="username" placeholder="Enter username">
<button type="submit">Submit</button>
</form>
</body>
</html>
These are just fundamental security measures – there are many more considerations to address.
Despite these challenges, PHP proved to be an interesting language to work with. I successfully implemented the Clean Architecture pattern and created a custom Inversion of Control container for dependency injection (as external libraries weren't permitted for this assignment). While PHP's weak and dynamic typing system has its drawbacks, it also offers flexibility that can be advantageous in certain scenarios.
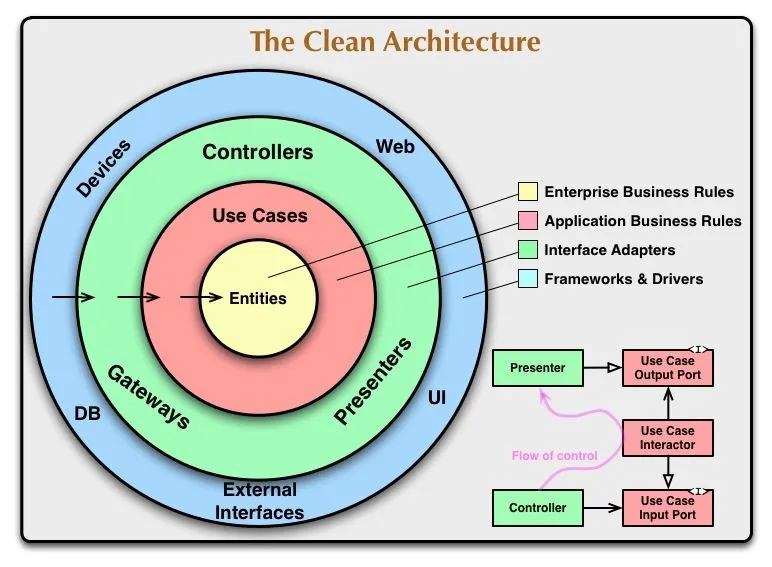
This experience gave me a deeper appreciation for the evolution of web development. Modern frameworks and libraries have significantly improved developer experience by handling many of these security concerns and architectural patterns out of the box, allowing developers to focus more on building features rather than implementing fundamental security measures.